ASP.NET MVC Coding Flow
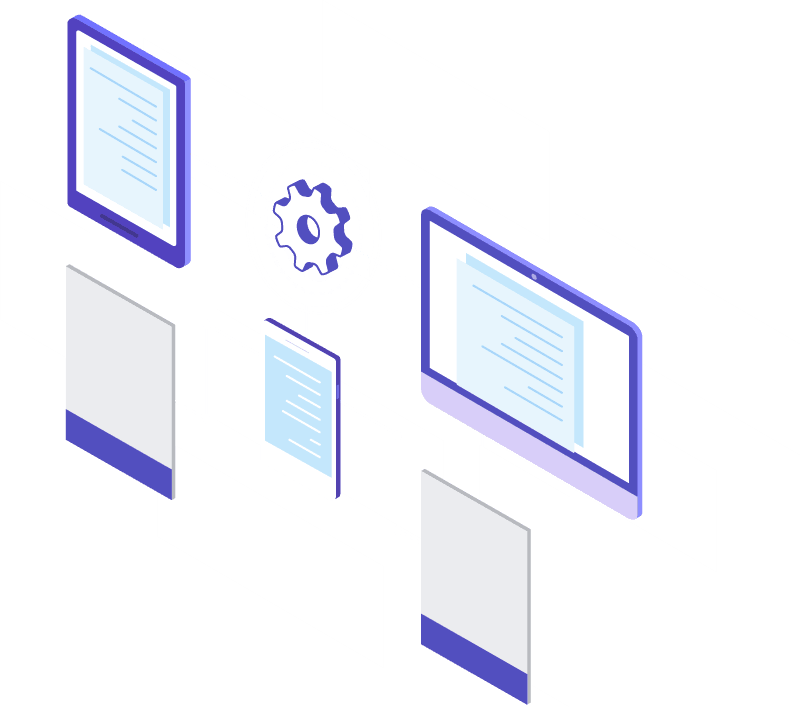
Step 1: Modify Model
This is the workflow we follow at Knowillence for ASP.NET MVC screen coding.
Open the model class in entity framework and add the decorators like the following to mark the validations and display details.
[Display(Name = “Start Date”)]
[DisplayFormat(DataFormatString = “{0:dd/MM/yyyy}”, ApplyFormatInEditMode = true)]
[Required]
Step 2: Controller Index Method
For index method add a condition to not show deleted records. We keep the following status for the records -1 for deleted, 0 for inactive and 1 for active. Add order by and order by some description field and not by ID when showing the list of records to the user.
public ActionResult Index()
{
var financialYears = db.FinancialYears.Include(f => f.RefRecordStatu).Where(rec => rec.RecordStatusID != LookupHelper.Deleted).OrderBy(rec => rec.FromDate);
return View(financialYears.ToList());
}
public class LookupHelper
{
public const int Deleted = -1;
public const int Inactive = 0;
public const int Active = 1;
}
Step 3: Controller Create Method – Get Action
For create method (get action) select Active as default record status.
public ActionResult Create()
{
ViewBag.RecordStatusID = new SelectList(db.RefRecordStatus, “RecordStatusID”, “RecordStatusDesc”, LookupHelper.Active);
return View();
}
Step 4: Controller Create Method – Post Action
For create method (post action) remove the unwanted fields from the binding list. Update the created by and created date.
public ActionResult Create([Bind(Include = “FinYearID,FinYear,FromDate,ToDate,FinYearCode,RecordStatusID”)] FinancialYear financialYear)
{
financialYear.CreatedBy = UserHelper.GetCurrentUserID();
financialYear.CreatedDate = DateTime.Now;
if (ModelState.IsValid)
{
db.FinancialYears.Add(financialYear);
db.SaveChanges();
return RedirectToAction(“Index”);
}
ViewBag.RecordStatusID = new SelectList(db.RefRecordStatus, “RecordStatusID”, “RecordStatusDesc”, financialYear.RecordStatusID);
return View(financialYear);
}
Step 5: Controller Edit Method – Post Action
For edit method (post action) remove the unwanted fields from the binding list. Update modified by and modified date. Skip any update to created by and created date.
public ActionResult Edit([Bind(Include = “FinYearID,FinYear,FromDate,ToDate,FinYearCode,RecordStatusID”)] FinancialYear financialYear)
{
financialYear.ModifiedBy = UserHelper.GetCurrentUserID();
financialYear.ModifiedDate = DateTime.Now;
if (ModelState.IsValid)
{
db.Entry(financialYear).State = EntityState.Modified;
db.Entry(financialYear).Property(x => x.CreatedDate).IsModified = false;
db.Entry(financialYear).Property(x => x.CreatedBy).IsModified = false;
db.SaveChanges();
return RedirectToAction(“Index”);
}
ViewBag.RecordStatusID = new SelectList(db.RefRecordStatus, “RecordStatusID”, “RecordStatusDesc”, financialYear.RecordStatusID);
return View(financialYear);
}
Step 6: Controller Delete Method – Post Action
For delete method post action set record status to -1 deleted and update modified by and modified date and save the record.
public ActionResult DeleteConfirmed(int id)
{
FinancialYear financialYear = db.FinancialYears.Find(id);
financialYear.RecordStatusID = LookupHelper.Deleted;
financialYear.ModifiedBy = UserHelper.GetCurrentUserID();
financialYear.ModifiedDate = DateTime.Now;
db.SaveChanges();
return RedirectToAction(“Index”);
}
Step 7: Views Index View
Set the view bag title correctly. add the following breadcrumb HTML code.
<div class=”section-header”>
<h1>Financial Years</h1>
<div class=”section-header-breadcrumb”>
<div class=”breadcrumb-item active”><a href=”#”>Order Settings</a></div>
<div class=”breadcrumb-item”><a href=”#”>Financial Year</a></div>
</div>
</div>
Display the table showing all the records inside this div. Remove the audit fields like created and modified and other unnecessary fields.
<div class=”section-body”>
<h2 class=”section-title”>
@Html.ActionLink(“Create New”, “Create”)
</h2>
<div class=”row”>
<div class=”card”>
<div class=”card-body”>
<table class=”table table-striped” id=”table-1″>
</table>
</div>
</div>
</div>
</div>
Step 8: Views Create and Edit View
Set the view bag title correctly. add the following breadcrumb HTML code.
<div class="section-header"> <div class="section-header"> <h1>Create a new financial year</h1> <div class="section-header-breadcrumb"> <div class="breadcrumb-item active"> <a href="#">Order Settings</a></div> <div class="breadcrumb-item"> @Html.ActionLink("Financial Years", "Index", "FinancialYears") </div> <div class="breadcrumb-item">Create</div> </div> </div> Enclose the HTML form within this div set. <div class="section-body"> <div class="row">
<div class="card col-md-8"> <div class="card-body">
</div> </div> </div></div>
Use multipart in the form if the user will be uploading a file.
@using (Html.BeginForm(“Create”, “Coupons”, FormMethod.Post, new { enctype = “multipart/form-data” }))
Choose the right editor types for the controls. Eg: for date use the HTML date picker.
@Html.TextBoxFor(model => model.FromDate, new
{
@type = “date”,
@class = “form-control datepicker”
})
The main action button class must be changed to btn-primary instead of btn-default.
Remove unwanted fields and created and modified audit fields.
Step 9: Views Detail and Delete View
Set the view bag title correctly. add the following breadcrumb HTML code.
<div class=”section-header”>
<h1>Delete financial year</h1>
<div class=”section-header-breadcrumb”>
<div class=”breadcrumb-item active”><a href=”#”>Order Settings</a></div>
<div class=”breadcrumb-item”>@Html.ActionLink(“Financial Years”, “Index”, “FinancialYears”)
</div>
<div class=”breadcrumb-item”>Delete</div>
</div>
</div>
Add the following heading before the main content for delete view.
<h3>Are you sure you want to delete this record?</h3>
Change the dl based list to table based list.
Place the table inside this div set.
<div class=”section-body”>
<div class=”row”>
<div class=”card col-md-6″>
<div class=”card-body”>
<div class=”form-horizontal”>
<table class=”table table-striped” id=”table-1″>
</table>
</div>
</div>
</div>
</div>
</div>
Change the primary action button class to btn-primary.
Keep the delete button form outside of the div set in the previous point.
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class=”form-actions no-color”>
<input type=”submit” value=”Delete” class=”btn btn-primary” /> |
@Html.ActionLink(“Back to List”, “Index”)
</div>
}
Step 10: Common Links
Ensure that Create, Edit, Delete, Details view has Back to List button at the bottom.
<div>
@Html.ActionLink(“Back to List”, “Index”)
</div>
Step 11: Controller Authorize Attribute
Add the Authorize attribute at the controller level if the screen has to be seen only after the user login to the portal. If the permission has to be given to specific roles for specific actions then mark Authorize attribute with Roles for each method in the controller.
H.Thirukkumaran
Founder & CEO
H.Thirukkumaran has over 20 years of experience in the IT industry. He worked in US for over 13 years for leading companies in various sectors like retail and ecommerce, investment banking, stock market, automobile and real estate He is the author of the book Learning Google BigQuery which explains how to build big data systems using Google BigQuery. He holds a masters in blockchain from Zigurat Innovation and Technology Business School from Barcelona Spain. He is also the India chapter lead for the Global Blockchain Initiative a non-profit from Germany that provides free education on blockchain. He currently lives in Chennai India.